将LangGraph与Ollama集成用于高级LLM应用
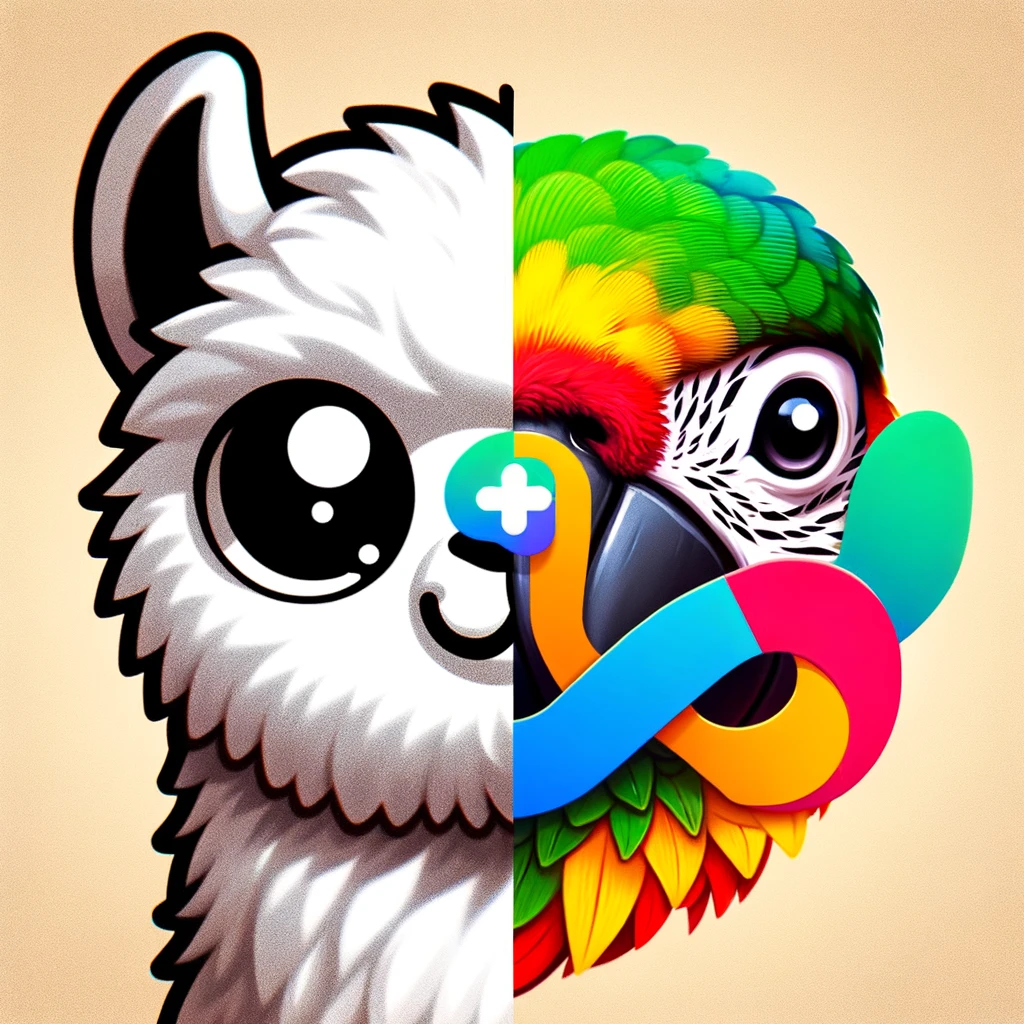
LangGraph 和 Ollama 是两个先进的库,当它们结合使用时,可以显著增强 Python 应用程序的能力,特别是在涉及有状态、多演员的应用领域。这篇博客文章将引导你如何利用这些工具创建更动态和响应式的应用程序。
LangGraph 和 Ollama 简介
LangGraph 是一个用于构建有状态、多演员应用的 Python 库。它基于 LangChain 并扩展了其功能,允许在循环的方式下协调多个链(或演员)在多个计算步骤中的交互。这对于向你的应用程序添加类似代理的行为特别有用,其中循环和周期对于维护状态并根据动态输入做出决策至关重要。
另一方面,Ollama 是 LangChain 生态系统的组成部分,专注于增强聊天模型和函数调用功能。它允许在应用程序中进行更微妙和上下文感知的交互,提供了一个强大的框架来处理复杂的对话流程。
通过将 LangGraph 与 Ollama 集成,开发人员可以利用两个库的优势创建复杂的应用程序,这些应用程序可以维护状态,处理复杂的交互,并根据用户输入和外部数据执行操作。
设置环境
首先,请确保您的 Python 环境中已安装 LangGraph 和必要的 LangChain 包。您还需要 Ollama 来处理聊天模型交互。使用 pip 进行安装:
pip install langgraph langchain langchain-community langchainhub langchain-core
ollama run openhermes
使用 LangGraph 和 Ollama 创建代理
我们示例的核心涉及设置一个可以响应用户查询的代理,例如提供当前时间。我们将使用 Ollama 处理聊天交互,并使用 LangGraph 维护应用程序的状态以及在不同操作之间管理流程。
定义代理状态和工具
定义您的代理状态及其可以使用的工具。在我们的示例中,我们使用一个简单的工具 get_now
来获取当前时间:
import operator
from datetime import datetime
from typing import Annotated, TypedDict, Union
from dotenv import load_dotenv
from langchain import hub
from langchain.agents import create_react_agent
from langchain_community.chat_models import ChatOllama
from langchain_core.agents import AgentAction, AgentFinish
from langchain_core.messages import BaseMessage
from langchain_core.tools import tool
from langgraph.graph import END, StateGraph
from langgraph.prebuilt import ToolExecutor, ToolInvocation
load_dotenv()
@tool
def get_now(format: str = "%Y-%m-%d %H:%M:%S"):
"""
Get the current time
"""
return datetime.now().strftime(format)
tools = [get_now]
tool_executor = ToolExecutor(tools)
集成 Ollama 并定义工作流
工具和状态定义好后,我们可以使用 LangGraph 设置 Ollama 和工作流:
class AgentState(TypedDict):
input: str
chat_history: list[BaseMessage]
agent_outcome: Union[AgentAction, AgentFinish, None]
intermediate_steps: Annotated[list[tuple[AgentAction, str]], operator.add]
model = ChatOllama(model="openhermes")
prompt = hub.pull("hwchase17/react")
agent_runnable = create_react_agent(model, tools, prompt)
def execute_tools(state):
print("Called `execute_tools`")
messages = [state["agent_outcome"]]
last_message = messages[-1]
tool_name = last_message.tool
print(f"Calling tool: {tool_name}")
action = ToolInvocation(
tool=tool_name,
tool_input=last_message.tool_input,
)
response = tool_executor.invoke(action)
return {"intermediate_steps": [(state["agent_outcome"], response)]}
def run_agent(state):
"""
#if you want to better manages intermediate steps
inputs = state.copy()
if len(inputs['intermediate_steps']) > 5:
inputs['intermediate_steps'] = inputs['intermediate_steps'][-5:]
"""
agent_outcome = agent_runnable.invoke(state)
return {"agent_outcome": agent_outcome}
def should_continue(state):
messages = [state["agent_outcome"]]
last_message = messages[-1]
if "Action" not in last_message.log:
return "end"
else:
return "continue"
workflow = StateGraph(AgentState)
workflow.add_node("agent", run_agent)
workflow.add_node("action", execute_tools)
workflow.set_entry_point("agent")
workflow.add_conditional_edges(
"agent", should_continue, {"continue": "action", "end": END}
)
workflow.add_edge("action", "agent")
app = workflow.compile()
input_text = "Whats the current time?"
运行代理
最后,你可以运行代理并与之交互。例如,你可以询问当前时间:
inputs = {"input": input_text, "chat_history": []}
results = []
for s in app.stream(inputs):
result = list(s.values())[0]
results.append(result)
print(result)
{'agent_outcome': AgentAction(tool='get_now', tool_input="format='%Y-%m-%d %H:%M:%S'", log="To get the current time, we can use the get_now tool.\nAction: get_now\nAction Input: format='%Y-%m-%d %H:%M:%S'")}
Called `execute_tools`
Calling tool: get_now
{'intermediate_steps': [(AgentAction(tool='get_now', tool_input="format='%Y-%m-%d %H:%M:%S'", log="To get the current time, we can use the get_now tool.\nAction: get_now\nAction Input: format='%Y-%m-%d %H:%M:%S'"), "format='2024-03-02 20:47:58'")]}
{'agent_outcome': AgentFinish(return_values={'output': 'The current time is March 02, 2024 20:47:58.'}, log='Final Answer: The current time is March 02, 2024 20:47:58.')}
{'input': 'Whats the current time?', 'chat_history': [], 'agent_outcome': AgentFinish(return_values={'output': 'The current time is March 02, 2024 20:47:58.'}, log='Final Answer: The current time is March 02, 2024 20:47:58.'), 'intermediate_steps': [(AgentAction(tool='get_now', tool_input="format='%Y-%m-%d %H:%M:%S'", log="To get the current time, we can use the get_now tool.\nAction: get_now\nAction Input: format='%Y-%m-%d %H:%M:%S'"), "format='2024-03-02 20:47:58'")]}
Final Answer: The current time is March 02, 2024 20:47:58
结论
通过将 LangGraph 与 Ollama 集成,Python 开发者可以创建出更交互性和响应性的应用程序。这个例子仅仅触及了可能性的表面。使用这些工具,您可以构建复杂的代理,能够处理各种任务,从客户服务机器人到复杂的数据分析工具。
记住,有效集成的关键在于理解每个组件的能力以及它们如何相互补充。通过实践和实验,您可以充分利用 LangGraph 和 Ollama 的全部功能,创建出真正创新的 Python 应用程序。